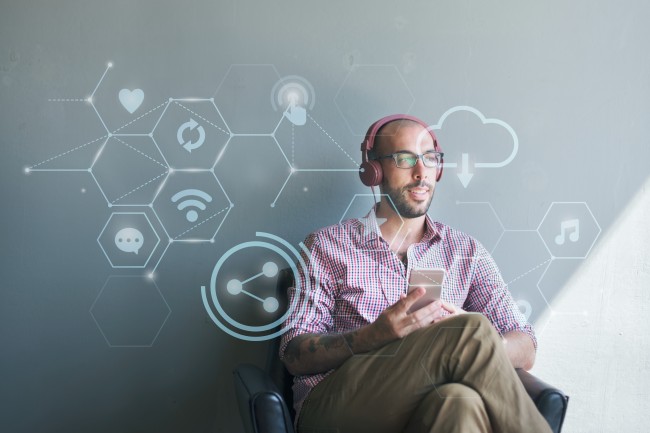
The Internet of Things (IoT) sounds fancy, but at its core, it’s about connecting devices to make life easier or more efficient. If you’ve ever wondered how your smart thermostat talks to your phone, you’re already thinking IoT.
Now, imagine building your own project. Whether you’re curious or starting a tech journey, this guide will help you create a simple IoT project using a Raspberry Pi. Let us get started.
Building Your First IoT Project
Step 1: Understanding the Basics of IoT
Before jumping into the project, let’s cover what IoT actually involves. IoT connects devices to share data, perform tasks, or provide insights. Think of it as a digital handshake between your gadgets. These devices can be anything—sensors, cameras, or even a coffee machine.
IoT projects usually involve three core components:
- Hardware: Physical devices like sensors or microcontrollers.
- Software: Code running on the device or in the cloud.
- Connectivity: The network that links everything together.
For your first project, you’ll stick to something straightforward: reading data from a temperature and humidity sensor and sending it to a cloud platform for monitoring.
Step 2: Gathering Your Tools and Materials
You don’t need a lot to start. A Raspberry Pi and a few basic components will get the job done. Here’s what you’ll need:
- Raspberry Pi (Any Model): Choose a Pi with Wi-Fi, like the Raspberry Pi 3 or 4. It’s your project’s brain.
- MicroSD Card (16GB or Larger): Acts as your Pi’s storage. Load it with the operating system.
- Power Supply for Raspberry Pi: Keep your Pi powered up.
- DHT11 or DHT22 Sensor: Measures temperature and humidity. The DHT22 is more accurate but costs slightly more.
- Jumper Wires: These connect the sensor to the Raspberry Pi.
- Breadboard: A board for wiring connections without soldering.
- Wi-Fi Connection: To send data to the cloud.
- A Laptop or Desktop: Used to set up the Raspberry Pi.
Optional but helpful:
- A case for your Raspberry Pi.
- A monitor, keyboard, and mouse for direct setup (though you can also set it up remotely).
Step 3: Setting Up Your Raspberry Pi
You’ll need to prepare the Raspberry Pi before adding any hardware. Here’s how to do it:
1. Download and Install Raspberry Pi OS
- Head to the official Raspberry Pi website and download the Raspberry Pi Imager tool.
- Insert the microSD card into your computer.
- Use the Imager tool to load Raspberry Pi OS (Lite is enough for this project) onto the card.
- Once done, insert the card into the Raspberry Pi.
2. Boot Your Raspberry Pi
- Power up your Pi using the micro-USB or USB-C power supply.
- If you’re connecting directly, attach a monitor, keyboard, and mouse.
- Alternatively, enable SSH for remote access:
- Create an empty file named
ssh
in the boot directory of your microSD card before inserting it into the Pi.
3. Connect to Wi-Fi
- If you’re using the Raspberry Pi Desktop, configure Wi-Fi in the GUI.
- For headless setups, edit the
wpa_supplicant.conf
file on the microSD card to include your Wi-Fi credentials.
4. Update and Install Essentials
Run the following commands in the terminal to update your Pi:
sudo apt update
sudo apt upgrade -y
Then, install Python and pip, which you’ll need for the project:
sudo apt install python3 python3-pip -y
Step 4: Connecting the Sensor
Now comes the fun part – hooking up your DHT sensor. Follow these steps to get it right:
1. Understand the Pins
The DHT11/DHT22 sensor has three pins:
- VCC: Connects to the Raspberry Pi’s 3.3V or 5V pin.
- GND: Connects to the ground pin.
- Data: Sends data to the Raspberry Pi.
2. Set It Up on the Breadboard
- Place the sensor on the breadboard.
- Use jumper wires to connect the sensor to the Raspberry Pi:
- Connect VCC to a 5V pin on the Raspberry Pi.
- Connect GND to a ground pin.
- Connect the Data pin to GPIO4 (Pin 7 on the Pi’s header).
3. Double-Check Connections
Check your wiring twice. Incorrect connections can damage the sensor or the Pi.
Step 5: Writing the Code
Once the hardware is set up, you’ll write the software to make it work.
1. Install Required Libraries
You’ll use the Adafruit DHT library to interact with the sensor. Run:
pip3 install Adafruit_DHT
2. Write the Python Script
Create a new Python file to read data from the sensor:
nano dht_sensor.py
Add this code:
import Adafruit_DHT
import time
# Define sensor type and GPIO pin
sensor = Adafruit_DHT.DHT11 # Use DHT22 if you have that sensor
pin = 4 # GPIO pin where the data line is connected
while True:
# Read temperature and humidity
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
print(f"Temp: {temperature:.1f}°C Humidity: {humidity:.1f}%")
else:
print("Failed to retrieve data from sensor")
# Wait before next reading
time.sleep(2)
3. Run the Script
Execute the script to see real-time temperature and humidity readings:
python3 dht_sensor.py
If everything’s wired correctly, you’ll see the data displayed in the terminal.
Step 6: Sending Data to the Cloud
Monitoring sensor data on your Raspberry Pi is great, but storing and viewing it remotely takes things up a notch. For this project, use ThingSpeak, a free cloud platform for IoT.
1. Set Up ThingSpeak
- Sign up at ThingSpeak.com.
- Create a new channel and add fields for temperature and humidity.
2. Install HTTP Library
Install the requests library to send data to ThingSpeak:
pip3 install requests
3. Modify Your Script
Update the Python script to send data to ThingSpeak:
import Adafruit_DHT
import requests
import time
sensor = Adafruit_DHT.DHT11
pin = 4
api_key = "YOUR_THINGSPEAK_API_KEY"
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
print(f"Temp: {temperature:.1f}°C Humidity: {humidity:.1f}%")
# Send data to ThingSpeak
url = f"https://api.thingspeak.com/update?api_key={api_key}&field1={temperature}&field2={humidity}"
response = requests.get(url)
if response.status_code == 200:
print("Data sent successfully")
else:
print("Failed to send data")
else:
print("Failed to retrieve data from sensor")
time.sleep(15) # ThingSpeak free tier limits to 15s intervals
Replace "YOUR_THINGSPEAK_API_KEY"
with your channel’s API key.
4. Run and Test
Run the script. Open your ThingSpeak channel to see the data visualized in real time.
Step 7: Expanding Your Project
Now that your first IoT device is working, consider enhancing it:
- Add more sensors for air quality, light levels, or motion detection.
- Use a battery pack to make it portable.
- Explore advanced platforms like AWS IoT or Google Cloud IoT.
Final Thoughts
Building your first IoT project might feel daunting, but you’ve taken the first step. With a Raspberry Pi and some basic components, you’ve created a functioning IoT device.
The beauty of IoT lies in experimentation and creativity. Start small, think big, and keep tinkering – you’ll be surprised by what you can create.