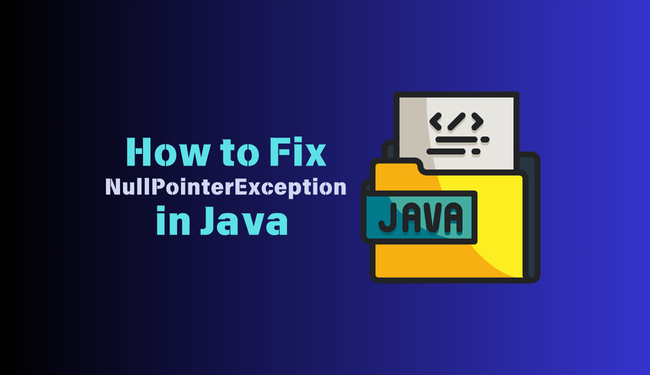
NullPointerException (NPE) is one of the most common exceptions that Java developers encounter. Understanding what causes NullPointerException, how to handle it, and how to avoid it can improve the coding practices and application stability.
Here in this guide we will cover everything you need to know about NullPointerException and help you catch and fix NullPointerException in Java.
What is NullPointerException in Java
NullPointerException is a runtime exception that occurs when Java application attempts to use an object reference that has not been initialized (i.e., it is null). When a method is invoked or a field is accessed on a null object, the Java Virtual Machine (JVM) throws a NullPointerException.
What Causes NullPointerException in Java
A NullPointerException can occur in different scenarios, most commonly it occurs when code tries to perform an operation on an object reference that has not been initialized.
Here are some common causes of NullPointerException in Java:
- Calling a Method on a Null Object: When you attempt to call a method on an object reference that is null, the JVM throws a NullPointerException.
- Accessing or Modifying a Field of a Null Object: If you try to access or modify a field of an object reference that is null, it results in a NullPointerException.
- Taking the Length of a Null Array: The error occurs when you try to take the length of an array that is null.
- Accessing or Modifying Elements of a Null Array: If you try to access or modify elements of an array that is null results in a NullPointerException.
- Throwing Null as If It Were a Throwable Value: If you attempt to throw null as if it were a Throwable value, it causes a NullPointerException.
- Unboxing Null Wrapper Objects: Unboxing a null wrapper object (e.g., converting an Integer object to an int primitive) results in a NullPointerException.
NullPointerException – Example
Let’s consider a real-world example to understand how a NullPointerException might occur in a Java application.
Example Scenario
Imagine you are developing a simple user registration system. Here is a class representing a User:
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
Now, you have a ‘UserService
‘ class that contains a method to register a user:
public class UserService {
public void registerUser(User user) {
if (user.getUsername().isEmpty()) {
throw new IllegalArgumentException("Username cannot be empty");
}
// More registration logic...
}
}
In the main
method, you attempt to register a new user:
public class Main {
public static void main(String[] args) {
UserService userService = new UserService();
User user = null; // This should be initialized properly
userService.registerUser(user); // This line will throw a NullPointerException
}
}
In the above code, the user
object is initialized to ‘null
‘. When userService.registerUser(user)
is called, the user
object is passed as ‘null
‘. Inside the registerUser
method, user.getUsername()
is invoked on a ‘null
‘ reference, leading to a ‘NullPointerException’.
How to Avoid NullPointerException
If you understand how to handle null values properly in Java than you can avoid ‘NullPointerException’ error in Java. Here are the best ways to avoid NullPointerException:
1. Initialize Objects Properly
Ensure that all objects are properly initialized before using them. So that you avoid referencing a null object.
User user = new User("JohnDoe", "password123");
2. Use Null Checks
Always check for ‘null’ before accessing methods or fields. Null check is a simple way to prevent NullPointerException.
if (user != null && user.getUsername() != null && !user.getUsername().isEmpty()) {
// Safe to use user.getUsername()
}
3. Use Optional Class
Java 8 introduced the ‘Optional
‘ class to handle null values. Optional
provides a container object which may or may not contain a non-null value.
Optional<String> username = Optional.ofNullable(user.getUsername());
username.ifPresent(name -> System.out.println("Username: " + name));
4. Use Ternary Operator
Use the ternary operator to provide a default value if an object is null. This can prevent null references by ensuring a fallback value.
String username = (user != null) ? user.getUsername() : "defaultUsername";
5. Use Apache Commons Lang
Apache Commons Lang library provides a utility class ‘StringUtils
‘ to handle null values safely.
if (StringUtils.isNotEmpty(user.getUsername())) {
// Safe to use user.getUsername()
}
6. Validate Inputs
Ensure that method parameters are validated before use to catch null values at the entry point of the method.
public void registerUser(User user) {
if (user == null) {
throw new IllegalArgumentException("User cannot be null");
}
if (user.getUsername() == null || user.getUsername().isEmpty()) {
throw new IllegalArgumentException("Username cannot be empty");
}
// More registration logic...
}
7. Use Annotations
Use annotations like @NonNull
to enforce non-null constraints in your code. Tools like Lombok can help automate this.
public void registerUser(@NonNull User user) {
// Method logic...
}
Avoiding NullPointerException – Example
Let’s rewrite the previous example to avoid NullPointerException using some of the strategies discussed above.
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
public class UserService {
public void registerUser(User user) {
if (user == null) {
throw new IllegalArgumentException("User cannot be null");
}
if (user.getUsername() == null || user.getUsername().isEmpty()) {
throw new IllegalArgumentException("Username cannot be empty");
}
// More registration logic...
}
}
public class Main {
public static void main(String[] args) {
UserService userService = new UserService();
User user = new User("JohnDoe", "password123");
userService.registerUser(user); // This will work without throwing NullPointerException
}
}
In the above code the ‘registerUser
‘ method first checks if the user
object is null and throws an ‘IllegalArgumentException
‘ if it is. It then checks if the username is null or empty before proceeding and ensure that ‘NullPointerException
‘ is avoided.
Conclusion
Don’t worry, NullPointerException is a very common issue in Java development. Just initialize the objects properly using null checks, use the Optional
class, validating inputs, and also use libraries and tools, to handle NullPointerExceptions.
Hope this article, helped you in avoiding NullPointerExceptions in Java applications. Thank you! Happy Coding.
Also Read: