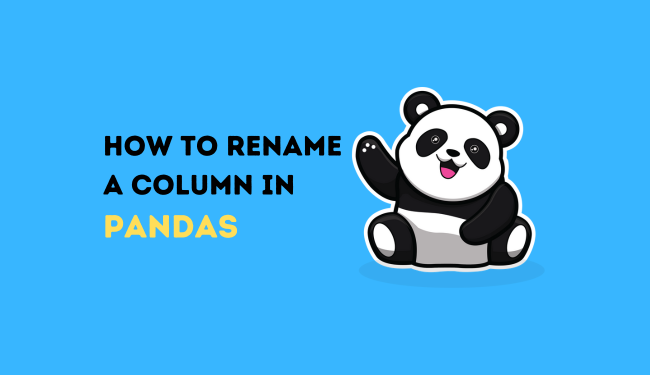
Hey there, data wrangler! Working with pandas DataFrames is always awesome, but sometimes you need to clean things up a bit. Sometimes it is common to rename columns to make the data more readable or to fit a specific format.
Here in this article, we’ll discuss on how to rename columns in pandas DataFrames using different methods. Let’s make this simple and fun. Ready? Let’s dive in!
Why Rename Columns?
First off, why bother renaming columns? Here are a few reasons:
- Readability: Clearer column names make your DataFrame easier to understand.
- Consistency: You might want to standardize column names across multiple DataFrames.
- Preparation: Preparing data for analysis often involves cleaning up column names.
Understanding the importance of clean and consistent column names helps you maintain organized and readable data.
How to Rename a Column in Pandas
Before getting started, make sure you have pandas installed. If you don’t have it yet, you can install it using pip:
pip install pandas
Now, let’s import pandas and create a sample DataFrame.
import pandas as pd
# Create a sample DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Great! Now we have a DataFrame to work with.
Method 1: Using rename(
)
The rename()
method is a flexible way to rename columns in a DataFrame. It allows you to rename specific columns by passing a dictionary where keys are the old column names and values are the new column names.
Example: Renaming Specific Columns
# Rename columns using a dictionary
df.rename(columns={'Name': 'Full Name', 'City': 'Location'}, inplace=True)
print(df)
Output:
Full Name Age Location
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Explanation:
- Dictionary Mapping: You create a dictionary with keys as old column names and values as new column names.
rename()
Method: Usedf.rename(columns=your_dict)
to rename columns.inplace=True
: Modify the DataFrame in place without needing to reassign it.
Method 2: Renaming All Columns
Sometimes, you need to rename all columns at once. You can achieve this by setting the columns
attribute directly.
Example: Renaming All Columns
# Rename all columns by assigning a new list to the columns attribute
df.columns = ['Name', 'Years', 'City']
print(df)
Output:
Name Years City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Explanation:
- New List of Column Names: Create a list of new column names.
- Assign to
columns
: Directly assign this list todf.columns
.
Method 3: Using set_axis()
The set_axis()
method is another way to rename all columns at once. It’s similar to setting the columns
attribute but a bit more versatile.
Example: Using set_axis()
# Rename all columns using set_axis
df.set_axis(['Person Name', 'Age in Years', 'City Name'], axis=1, inplace=True)
print(df)
Output:
Person Name Age in Years City Name
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Explanation:
- New List of Column Names: Create a list of new column names.
set_axis()
Method: Usedf.set_axis(new_columns, axis=1, inplace=True)
to rename columns.
Method 4: Renaming Columns Using a Function
You might want to rename columns by applying a function to each column name. This is useful for standardizing column names or making them lowercase.
Example: Applying a Function to Column Names
# Function to modify column names
def modify_column(col):
return col.lower().replace(' ', '_')
# Apply function to column names
df.columns = [modify_column(col) for col in df.columns]
print(df)
Output:
person_name age_in_years city_name
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Explanation:
- Function Definition: Define a function that takes a column name and returns the modified name.
- List Comprehension: Use a list comprehension to apply the function to each column name.
- Assign to
columns
: Assign the resulting list todf.columns
.
Practical Tips for Renaming Columns
Here are some tips to make the most of renaming columns in pandas:
- Be Descriptive: Use clear and descriptive column names.
- Stay Consistent: Keep a consistent naming convention across your DataFrames.
- Use Inplace: Use
inplace=True
to modify the DataFrame directly if you don’t want to reassign it.
Example: Combining Tips
# Rename columns to be more descriptive and consistent
df.rename(columns={'person_name': 'full_name', 'age_in_years': 'age', 'city_name': 'city'}, inplace=True)
print(df)
Output:
full_name age city
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Using add_prefix()
and add_suffix()
Pandas also provides add_prefix()
and add_suffix()
methods to add a prefix or suffix to all column names. This can be useful for distinguishing columns after merging DataFrames.
Example: Adding Prefix
# Add prefix to column names
df_prefixed = df.add_prefix('data_')
print(df_prefixed)
Output:
data_full_name data_age data_city
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Example: Adding Suffix
# Add suffix to column names
df_suffixed = df.add_suffix('_info')
print(df_suffixed)
Output:
full_name_info age_info city_info
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Explanation:
add_prefix()
Method: Usedf.add_prefix('prefix_')
to add a prefix to all column names.add_suffix()
Method: Usedf.add_suffix('_suffix')
to add a suffix to all column names.
Advanced Column Renaming with str.replace()
For more advanced renaming tasks, you can use the str.replace()
method to apply regular expressions to column names.
Example: Using str.replace()
# Create a DataFrame with complex column names
data = {
'First Name': ['Alice', 'Bob', 'Charlie'],
'Last Name': ['Smith', 'Johnson', 'Williams'],
'City of Residence': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
# Use str.replace to rename columns
df.columns = df.columns.str.replace(' ', '_').str.lower()
print(df)
Output:
first_name last_name city_of_residence
0 Alice Smith New York
1 Bob Johnson Los Angeles
2 Charlie Williams Chicago
Explanation:
- Complex Column Names: Create a DataFrame with complex column names.
str.replace()
Method: Usedf.columns.str.replace(' ', '_').str.lower()
to replace spaces with underscores and convert to lowercase.
Conclusion
Renaming columns in pandas DataFrames is a fundamental task for any data professional. Whether you need to rename specific columns, all columns, or apply a function to each column name, pandas offers multiple ways to get the job done.
From using the rename()
method and setting the columns
attribute to advanced techniques like str.replace()
, you now have a toolkit full of options to clean and organize your DataFrames.
Remember, clear and consistent column names make your data more readable and easier to work with. So take the time to rename your columns thoughtfully. Happy coding, and may your DataFrames always be well-named!
Also Read: