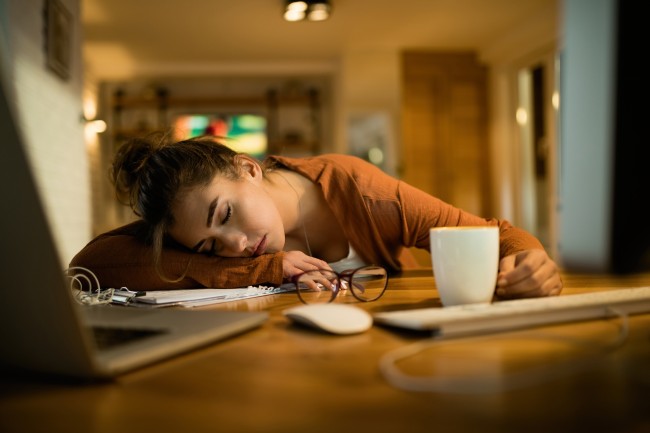
So, you’re in the world of JavaScript and wondering how to make your code take a break, huh? Let’s discuss about the sleep function in JavaScript.
This isn’t your regular nap; it’s about pausing code execution. It’s a bit tricky because JavaScript doesn’t have a built-in sleep function like some other languages. But don’t worry, we’ll figure it out together. Ready? Let’s get started!
Why Would You Need a Sleep Function?
Before we jump into the how, let’s talk about the why. Imagine you’re building a fancy web app, and you need to:
- Wait for an animation to complete before doing the next thing.
- Throttle API requests to avoid overwhelming the server.
- Simulate a delay for testing purposes.
In these cases, a sleep function is super handy. It pauses your code, making it wait before moving on to the next task. But remember, in JavaScript, it’s more about asynchronous behavior than actually sleeping.
Implementing Sleep Function in JavaScript?
JavaScript is single-threaded, meaning it can only do one thing at a time. But, thanks to its asynchronous capabilities, it can juggle multiple tasks without freezing up.
The key players here are callbacks, promises, and async/await. These let you manage time-consuming tasks without blocking the main thread.
The Classic SetTimeout Trick
Alright, let’s get to it. The simplest way to make JavaScript “sleep” is by using setTimeout
. This function lets you run a piece of code after a specified delay.
Here’s a quick example:
console.log('Start');
setTimeout(() => {
console.log('Pause for 2 seconds');
}, 2000);
console.log('End');
When you run this, you’ll see “Start” and “End” immediately, then “Pause for 2 seconds” after, well, 2 seconds. It doesn’t actually stop the code; it just schedules the delayed code to run later.
Creating a Sleep Function with Promises
‘setTimeout
‘ is nice, but it doesn’t quite give you that sleep feeling. For that, we need promises. Promises allow us to handle asynchronous operations more cleanly. Let’s create a sleep function using promises.
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
With this function, you can write code like this:
console.log('Start');
sleep(2000).then(() => {
console.log('Pause for 2 seconds');
});
console.log('End');
But, this still looks a bit messy. You have to chain ‘then
‘ calls, which can get ugly in complex scenarios.
Using Async/Await for Cleaner Code
Async/await to the rescue! Introduced in ES2017, async/await makes your asynchronous code look synchronous, which is easier to read and maintain.
First, you mark a function as async
. Then, inside that function, you can use await
to pause execution until a promise resolves.
Here’s our sleep function in action with async/await:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function demoSleep() {
console.log('Start');
await sleep(2000);
console.log('Pause for 2 seconds');
console.log('End');
}
demoSleep();
Now it looks much neater, right? The ‘await
‘ keyword makes JavaScript wait for the promise from sleep
to resolve before moving on. This makes your code easy to understand.
Practical Applications of Sleep in JavaScript
Let’s see some real-world scenarios where this sleep function comes in handy.
1. Throttling API Requests
If you’re working with APIs, you know servers have rate limits. Exceeding these can get you blocked. To avoid this, you can space out your requests.
async function fetchWithPause(urls) {
for (const url of urls) {
const response = await fetch(url);
const data = await response.json();
console.log(data);
await sleep(1000); // Pause for 1 second between requests
}
}
const urls = ['https://api.example.com/data1', 'https://api.example.com/data2'];
fetchWithPause(urls);
2. Animations and User Experience
Maybe you’re building a game or a fancy UI and need to wait between animations. Sleep can help here too.
async function animateSteps(steps) {
for (const step of steps) {
console.log(`Animating: ${step}`);
// Imagine some animation code here
await sleep(500); // Pause for 0.5 seconds between steps
}
}
const steps = ['Step 1', 'Step 2', 'Step 3'];
animateSteps(steps);
3. Simulating Delays in Tests
Testing sometimes requires simulating delays to see how your code handles them. Sleep can make this simple.
async function runTest() {
console.log('Test started');
await sleep(3000); // Simulate a 3-second delay
console.log('Test completed');
}
runTest();
Common Pitfalls and Best Practices
While adding sleep to your JavaScript toolkit, be mindful of a few things:
- Don’t overuse sleep: It can make your code less responsive. Use it sparingly and only when necessary.
- Avoid blocking the main thread: JavaScript is single-threaded, and blocking the main thread can make your app unresponsive. Stick to asynchronous patterns.
- Error handling: Always handle errors, especially when working with promises. You don’t want an unhandled promise rejection to crash your app.
Here’s an improved version of our sleep function with error handling:
function sleep(ms) {
return new Promise((resolve, reject) => {
if (typeof ms !== 'number' || ms < 0) {
reject(new Error('Invalid milliseconds value'));
} else {
setTimeout(resolve, ms);
}
});
}
// Usage with async/await
(async () => {
try {
console.log('Start');
await sleep(2000);
console.log('Pause for 2 seconds');
console.log('End');
} catch (error) {
console.error('Error:', error);
}
})();
This ensures your code is robust and can handle unexpected inputs gracefully.
Final Thoughts
Implementing a sleep function in JavaScript is a neat trick that can make your code more effective in certain situations. While JavaScript doesn’t provide a native sleep function, using ‘setTimeout
‘, promises, and async/await, you can achieve the desired effect with ease.
So next time you need to pause your code, remember these tricks. They’ll help you manage time-consuming tasks without turning your code into a mess. Happy coding, and may your JavaScript dreams be filled with perfectly timed pauses!
Also Read: