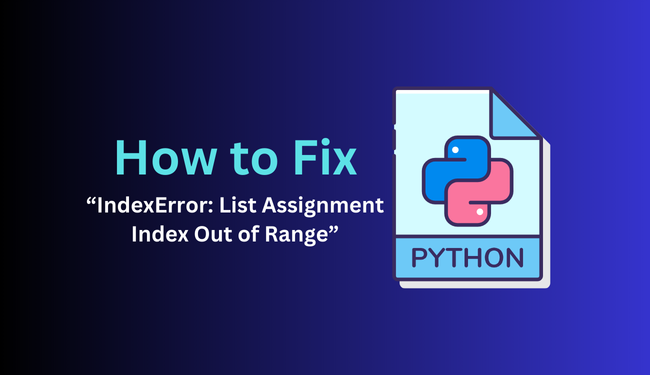
When working on lists in Python, one of the most common errors that occur is the ‘IndexError: list assignment index out of range
‘. The error indicates that you are trying to access or assign a value to an index that is not currently available in the list.
Understanding why ‘IndexError: list assignment index out of range
‘ occur is very important for developers to write error-free code.
Contents
Understanding List Indexing in Python
In Python, lists are ordered collections that allow you to store and manipulate a sequence of elements. Each element in a list is associated with an index, starting from 0.
For example, in a list with three elements [10, 20, 30]
, the indices are 0
, 1
, and 2
respectively. Attempting to access or modify an index outside of this range, such as 3
in this case, results in an ‘IndexError
‘.
Why “IndexError: List Assignment Index Out of Range” occur
- Accessing Non-Existent Indices: When developers try to access an index that does not exist in the list. For instance, trying to access
my_list[3]
whenmy_list
has only three elements. - Assigning Values to Out-of-Range Indices: Try to assign a value to an index that is beyond the current length of the list. This often happens when programmers mistakenly assume the list has more elements than it actually does.
- Looping Beyond List Length: Using loops that iterate beyond the length of the list, especially in scenarios where list elements are modified within the loop, leading to unexpected index ranges.
- Dynamic List Expansion Errors: Incorrectly expanding a list by attempting to assign values to indices that have not been initialized, rather than using methods that safely add elements to the list.
Example of “IndexError: List Assignment Index Out of Range”
Here is an example that show the ‘IndexError
‘ message when running the code.
Example:
# Initial list with 3 elements
my_list = [10, 20, 30]
# Attempt to assign a value to the fourth element (index 3)
my_list[3] = 40
Output
When you run the above code, you will encounter the following error message:
Traceback (most recent call last):
File "example.py", line 5, in <module>
my_list[3] = 40
IndexError: list assignment index out of range
How to resolve “IndexError: List Assignment Index Out of Range”
To fix the ‘IndexError: list assignment index out of range
‘ error in Python you must ensure that you only access or assign values to indices that exist within the list. Here are several strategies to fix this error:
1. Ensure the Index Exists
Before assigning a value to a specific index, make sure the index is within the bounds of the list.
Example:
my_list = [10, 20, 30]
index = 3
value = 40
if index < len(my_list):
my_list[index] = value
else:
print(f"Index {index} is out of range. List length is {len(my_list)}.")
2. Append to the List
If you want to add a new element to the end of the list and avoid out-of-range assignments, use the append
method.
my_list = [10, 20, 30]
# Correct way to add a new element
my_list.append(40)
print(my_list) # Output: [10, 20, 30, 40]
3. Extend the List
Add multiple elements or ensure a specific index exists, extend the list with ‘None'
or default values to accommodate the new elements.
my_list = [10, 20, 30]
# Ensure the list is long enough to assign a value at index 5
index = 5
value = 50
if index >= len(my_list):
my_list.extend([None] * (index - len(my_list) + 1))
my_list[index] = value
print(my_list) # Output: [10, 20, 30, None, None, 50]
4. Use List Comprehension for Dynamic List Creation
Dynamically create a list based on a certain size, you can use list comprehension to initialize the list with default values.
# Create a list of size 6 initialized with 0s
size = 6
my_list = [0] * size
# Assign values within the list bounds
for i in range(size):
my_list[i] = i * 10
print(my_list) # Output: [0, 10, 20, 30, 40, 50]
5. Use Try-Except Block
For better handling, especially in large programs where list operations are more complex, use a try-except block to catch and handle the ‘IndexError
‘.
my_list = [10, 20, 30]
try:
index = 4
value = 40
my_list[index] = value
except IndexError as e:
print(f"Error: {e}. You tried to access index {index}, but the list length is {len(my_list)}.")
Final Thoughts:
To fix the “IndexError: list assignment index out of range
“, follow these best practices:
- Check Index Bounds: Always ensure the index is within the bounds of the list.
- Append for New Elements: Use
append
to add new elements to the end of the list. - Extend List Size: Use
extend
to increase the list size if necessary. - Initialize Lists Properly: Use list comprehension or other methods to create lists with the desired size.
- Error Handling: Use try-except blocks for robust error handling.
By following these practices, you can avoid and resolve the ‘IndexError: list assignment index out of range
‘ in your Python programs.
Also Read: