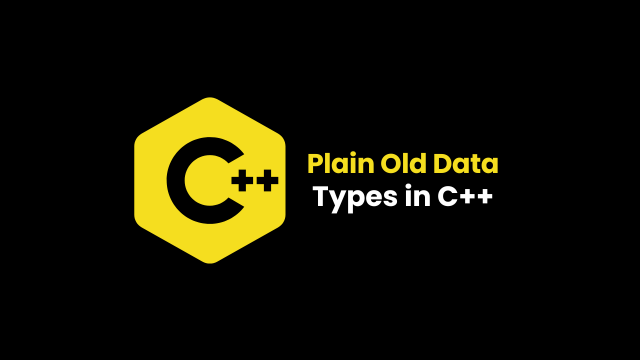
If you’re into C++ programming, you probably know the term “Plain Old Data” or POD types. It sounds a bit like an odd relic from the past, right? Well, it kind of is – but in the best way possible.
Let us break it down for you, step by step, so you can see why understanding POD types is actually super important when you’re coding in C++.
Contents
What Exactly Are POD Types?
Think of POD types as the simple data structures in C++. A POD type is a class or struct that is compatible with C-style data structures. If you’ve used C, you’ll recognize that this means no hidden complexities or dynamic behavior.
They behave predictably in memory, making them easy to use when performance is crucial, like when you’re working with low-level hardware or systems programming.
Example of a POD Type
#include <iostream>
struct Point {
int x;
int y;
}
int main() {
Point p1;
p1.x = 10;
p1.y = 20;
// Accessing and printing values
std::cout << "Point coordinates: (" << p1.x << ", " << p1.y << ")" << std::endl;
return 0;
}
Why is this a POD?
- No Constructors or Destructors: The
Point
struct doesn’t define any constructors or destructors. When an instance ofPoint
is created, its memory is allocated, but the members (x
andy
) aren’t automatically initialized. - No Virtual Functions: There’s no use of polymorphism, so no virtual functions exist. Everything is plain and static.
- Public Members Only: The variables
x
andy
are public, meaning they can be accessed and modified directly from outside the struct. - No Inheritance: The struct doesn’t inherit from any other class or struct.
- Trivially Copyable: You can copy or assign instances of
Point
using the default copy behavior provided by the compiler:
Key Characteristics of POD Types
Okay, let’s get down to the nitty-gritty. What makes a class or struct a POD? It boils down to a few specific rules that the structure has to follow:
- No Constructors or Destructors: A POD can’t have any custom constructors or destructors. This means when an instance of the class is created or destroyed, C++ won’t do anything behind the scenes. Everything is just as you’d expect—nothing gets initialized automatically, and nothing fancy happens when it goes out of scope.
- No Virtual Functions: If you’ve ever dealt with virtual functions or polymorphism in C++, you know how powerful they can be. But PODs don’t allow them. That’s because virtual functions introduce dynamic behavior, which contradicts the static, predictable nature of PODs.
- Public Members Only: All member variables in a POD struct or class are public. There’s no encapsulation or hiding of data. Everything is out in the open—easy to access, easy to modify.
- No Inheritance: PODs can’t inherit from other classes or structs. They stand alone. This lack of inheritance ensures there’s no extra baggage to deal with—no complex hierarchies or hidden base class members.
- Trivially Copyable and Assignable: This is a big one. POD types can be copied and assigned without the need for any special functions. The default behavior provided by the compiler is exactly what you want. Nothing extra happens—just a simple bitwise copy.
By now, you might be thinking, “Wow, these POD types are really bare-bones.” And you’d be right! But that’s exactly their charm. There’s no magic, no hidden complexity—what you see is what you get.
Why Should You Care About POD Types?
You’re probably wondering, “Why does any of this matter? C++ has more modern features, so why should I bother with POD types?” Here’s where things get interesting.
First off, performance. In certain scenarios – especially in systems programming or game development – every microsecond counts. POD types, because they’re so simple, allow for incredibly fast memory operations.
They can be copied, moved, and stored efficiently without any overhead. In fact, they behave almost like raw data in memory. So, if speed is a concern, POD types can be your best friend.
Second, compatibility. POD types can be shared between C++ and C codebases easily. Since they don’t rely on any fancy C++-only features, they maintain compatibility with older C code.
If you ever find yourself working on a project that mixes C and C++, or working with low-level APIs that require C-compatible data structures, PODs are invaluable.
Lastly, predictability. When you’re debugging or working with low-level code, the last thing you want is unpredictable behavior. POD types give you that predictability. Their behavior is simple, making them much easier to understand and debug compared to more complex C++ classes with constructors, destructors, and virtual functions.
When Should You Use POD Types?
Now, I’m not saying you should use POD types for everything. C++ gives you plenty of modern tools for a reason. But there are definitely situations where a good old POD type makes perfect sense:
- Interfacing with C libraries: Since POD types are compatible with C, they’re the perfect choice when you’re working with external C libraries. No need to worry about C++ features that C doesn’t support—just clean, compatible data.
- Performance-Critical Applications: Whether you’re writing a high-performance game engine or some low-level system code, POD types can give you that extra performance boost. Their memory layout is predictable, and operations like copying are lightning-fast.
- Serialization and Network Code: When you’re serializing data or working with network protocols, POD types are ideal because their memory layout is straightforward. You know exactly how they’re represented in memory, making it easy to send them over the network or write them to a file.
The Downside of POD Types
Of course, nothing’s perfect, and POD types come with their own set of limitations. The biggest downside is that they’re, well, plain. You lose out on a lot of the powerful features that C++ offers, like encapsulation, inheritance, and constructors that manage resources.
If you’re working on a larger, more complex project, using nothing but POD types could lead to messy, unstructured code. You’d have to manually handle initialization, resource management, and data protection, which can make your code more error-prone.
So, while POD types are great for certain use cases, they’re not a magic bullet. You’ll need to weigh the benefits of simplicity and performance against the need for more structured, feature-rich classes.
Conclusion:
So, there you have it. Plain Old Data types in C++ might not be the flashiest tool in your programming toolbox, but they’re solid, reliable, and sometimes exactly what you need.
Whether you’re chasing performance, working with C libraries, or just a simple data structure, POD types are worth knowing about.
Also Read: