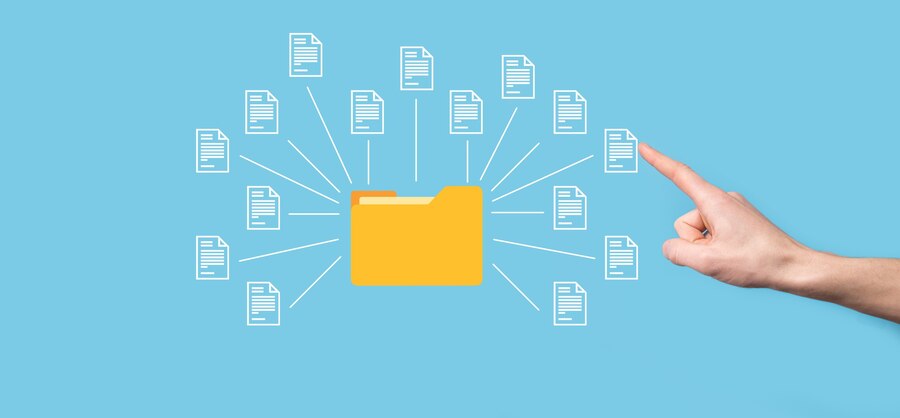
If you’ve ever worked on a project that requires compiling code, you know it can get messy. Different files, multiple dependencies, and repeated commands can quickly turn into a headache.
Enter Makefile, the unsung hero of automation in programming. Whether you’re a beginner or a seasoned coder, Makefiles are worth having in your toolkit. Here in this article, we will discuss what a Makefile is, how it works, and how you can use it to simplify your workflow.
What is a Makefile?
A Makefile is like a to-do list for your computer. It tells your system what tasks to run and in what order. It’s a simple text file that defines a set of rules or instructions, typically for compiling and linking programs. Instead of typing a dozen commands to build your project, you just run make
, and it handles the heavy lifting.
The tool behind it, called make
, reads the Makefile and decides what needs to be done. If you’re working on a project with source files in C, C++, or another compiled language, Makefiles save time by automating repetitive tasks.
But it’s not just for compiling – Makefiles can handle any task you can describe with commands, from cleaning up temporary files to running tests.
How Does a Makefile Work?
At its core, a Makefile is made up of rules. Each rule tells make
how to create a target. Targets are usually files, like executables or object files, but they can also be abstract tasks. Each rule has three parts:
- Target: The name of the output file or task.
- Dependencies: The files or inputs required to create the target.
- Commands: The shell commands to build the target.
Here’s a basic example to illustrate:
hello: hello.o utils.o
gcc -o hello hello.o utils.o
hello.o: hello.c
gcc -c hello.c
utils.o: utils.c
gcc -c utils.c
When you run make hello
, make
checks the dependencies. If hello.o
or utils.o
are missing or outdated, it builds them. Then, it links everything to create hello
. It’s like a chain reaction, where make
only updates what’s necessary.
Why Should You Use a Makefile?
Without Makefiles, you’d need to remember every file and dependency in your project. One typo or forgotten command could waste hours debugging. With Makefiles, everything is documented and automated. They’re reliable, repeatable, and save you from reinventing the wheel every time you build your project.
Beyond saving time, Makefiles also keep projects consistent across teams. If everyone uses the same Makefile, nobody has to guess how to build or run the program. It’s especially helpful in open-source projects or when sharing code.
Basic Makefile Examples
Let’s start with a simple example to compile a single C program. Say you have a file called main.c
. Normally, you’d run:
gcc -o main main.c
Here’s how a Makefile simplifies this:
main: main.c
gcc -o main main.c
Now, typing make
in the terminal runs that command. But wait—it gets better. What if your program grows to include multiple files? A Makefile can handle that too:
program: main.o file1.o file2.o
gcc -o program main.o file1.o file2.o
main.o: main.c
gcc -c main.c
file1.o: file1.c
gcc -c file1.c
file2.o: file2.c
gcc -c file2.c
Whenever you edit a file, make
automatically knows what to rebuild. It skips unnecessary steps, so it’s faster than running everything manually.
Advanced Features of Makefiles
Makefiles aren’t just about compiling code. They come with powerful features that let you handle complex projects with ease. Let’s explore a few:
1. Variables
Variables let you avoid repeating yourself. For instance, if you’re using the same compiler flags everywhere, define a variable for them:
CC = gcc
CFLAGS = -Wall -g
program: main.o file1.o file2.o
$(CC) $(CFLAGS) -o program main.o file1.o file2.o
%.o: %.c
$(CC) $(CFLAGS) -c $<
Here, $(CC)
and $(CFLAGS)
are replaced with their values. It’s cleaner, easier to read, and simple to update.
2. Pattern Rules
Pattern rules let you define how to build multiple files without writing separate rules for each one. The %
acts as a wildcard:
%.o: %.c
gcc -c $<
This rule tells make
how to build any .o
file from a .c
file. The $<
is a shorthand for the first dependency (in this case, the .c
file).
3. Phony Targets
Not all targets are files. Sometimes, you need tasks like cleaning up temporary files or running tests. For these, you use phony targets:
.PHONY: clean test
clean:
rm -f *.o program
test:
./run_tests.sh
Phony targets don’t create files – they’re just placeholders for commands.
Advanced Patterns and Functions
Makefiles also support built-in functions for string manipulation, file checks, and more. Let’s look at a few:
1. Automatic Dependency Generation
Keeping track of dependencies by hand is tedious. You can automate it with a rule like this:
%.d: %.c
gcc -MM $< > $@
include $(wildcard *.d)
Here, gcc -MM
generates a .d
file listing dependencies for each .c
file. The include
directive loads these .d
files, so make
knows what to rebuild.
2. Conditional Execution
Sometimes, you need different behavior depending on your environment. Use conditionals to make your Makefile smarter:
ifeq ($(OS),Windows_NT)
RM = del
else
RM = rm -f
endif
clean:
$(RM) *.o program
This snippet sets the RM
command based on your operating system.
3. Recursive Makefiles
For large projects, it’s common to divide the work into multiple Makefiles. A top-level Makefile can call others:
all: lib1 lib2
lib1:
$(MAKE) -C lib1
lib2:
$(MAKE) -C lib2
The -C
option tells make
to run in a different directory.
Common Pitfalls and Tips
- Always Use Tabs: Makefiles require tabs for indentation, not spaces. This trips up even experienced developers.
- Organize Rules Clearly: Group related rules and use comments generously. A messy Makefile is hard to maintain.
- Test Your Makefile: Run it after every change to catch mistakes early.
Wrapping Up
Makefiles might look intimidating at first, but they’re easier than you think. Once you start using them, you’ll wonder how you ever lived without them.
They save time, reduce errors, and bring order to chaos. Whether you’re working on a personal project or contributing to open-source, mastering Makefiles is a skill worth having.
So why not create your first Makefile today? Start simple, and as your projects grow, you can layer on advanced features. Before you know it, you’ll be automating tasks like a pro. Happy coding!